Blog
Messaging
Blog
Blog
Contact Center
Security
Spoofing, decoded: What it is, and how to stop it from sneaking into your contact center
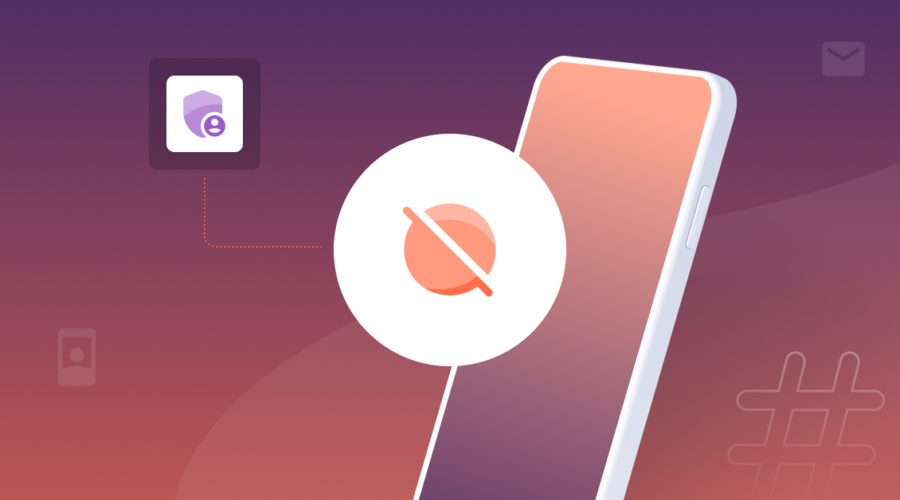
Blog
Messaging
SMS for Edtech platforms
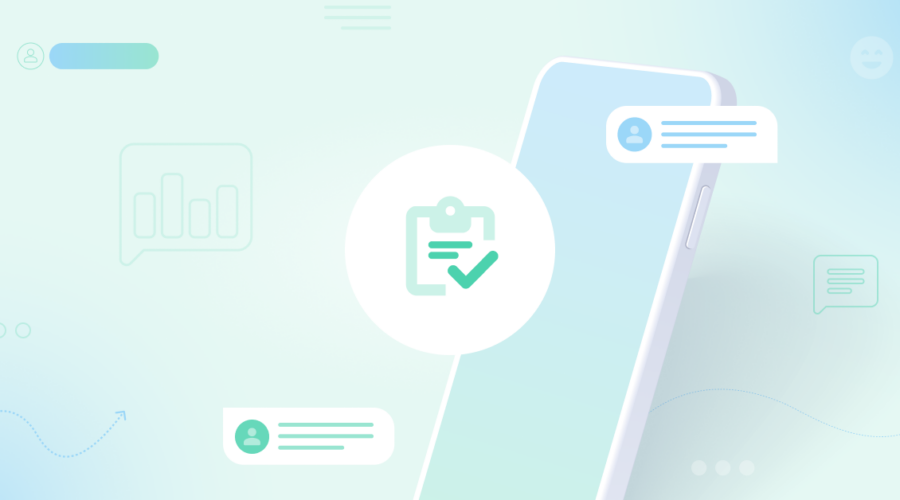
Blog
Contact Center
The missing piece to your contact center’s business continuity strategy
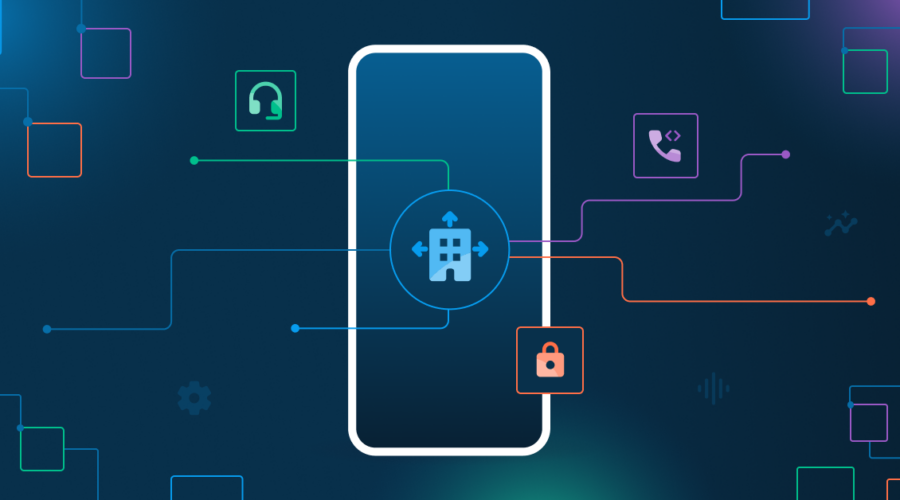
Blog
Messaging
What we said, and heard, at MEF Barcelona
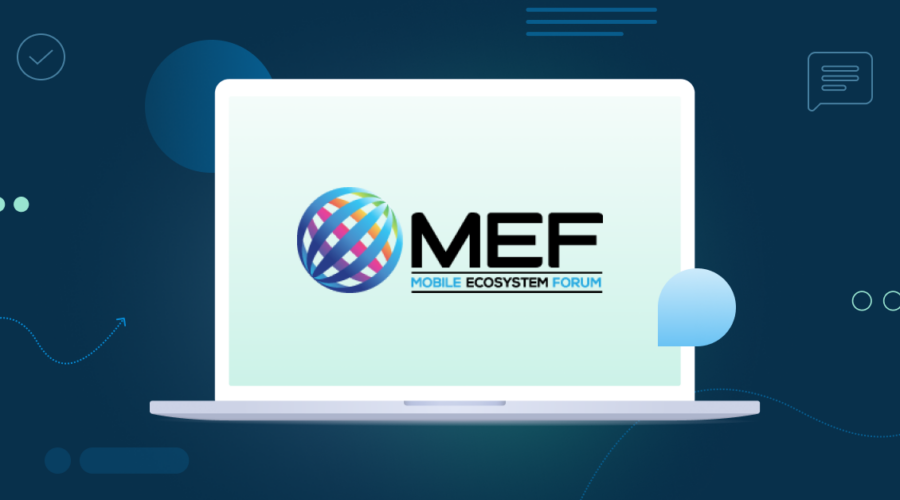
Blog
Messaging
Campaign drift in business texting
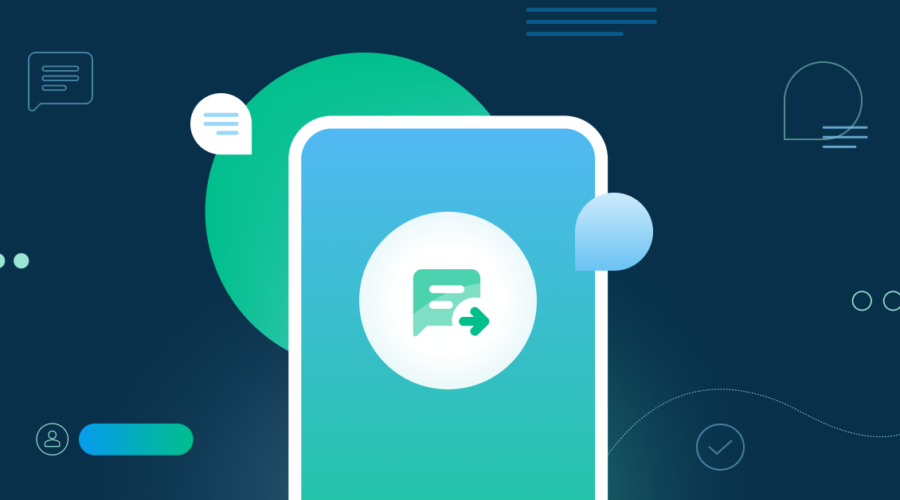
Blog
Messaging
Taking your message global in 2024?
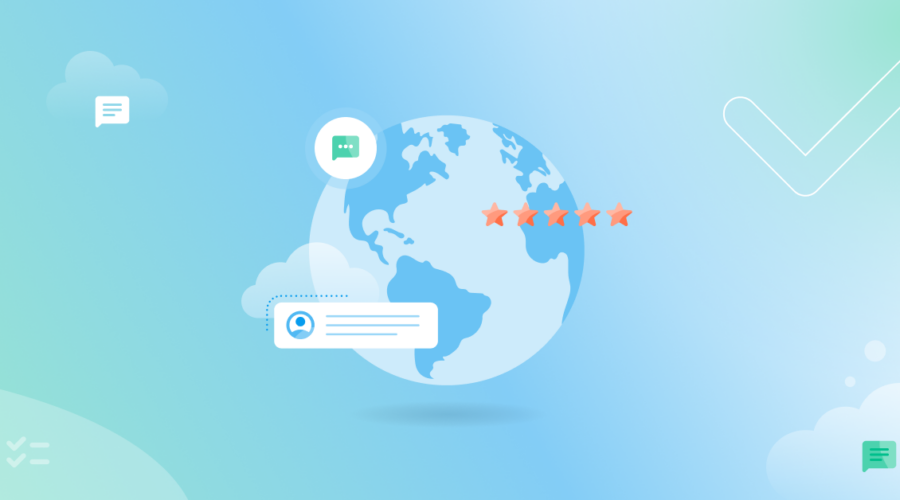