Quick Guide
Voice
Resources
Explore our guides, reports, webinars, and more.
Quick Guide
Messaging
SMS deliverability checklist
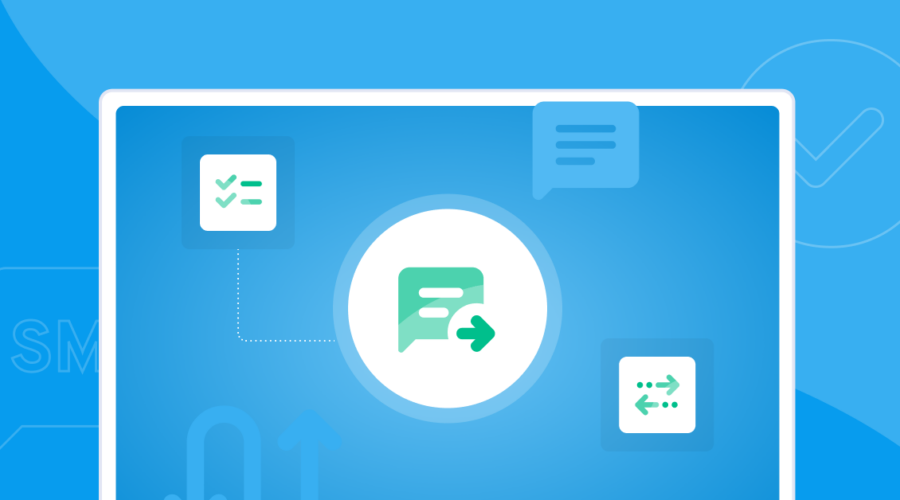
Quick Guide
Avoid migration migraines with Bandwidth
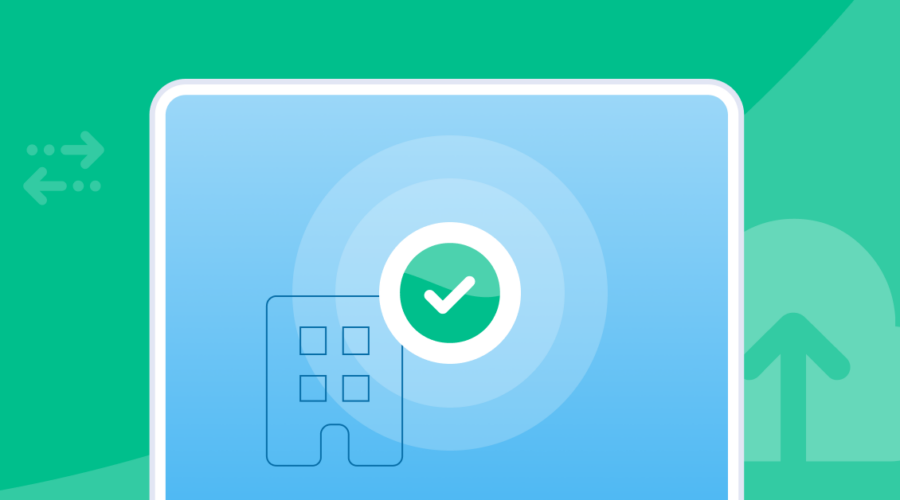
Research Report
Enterprise Communications Landscape
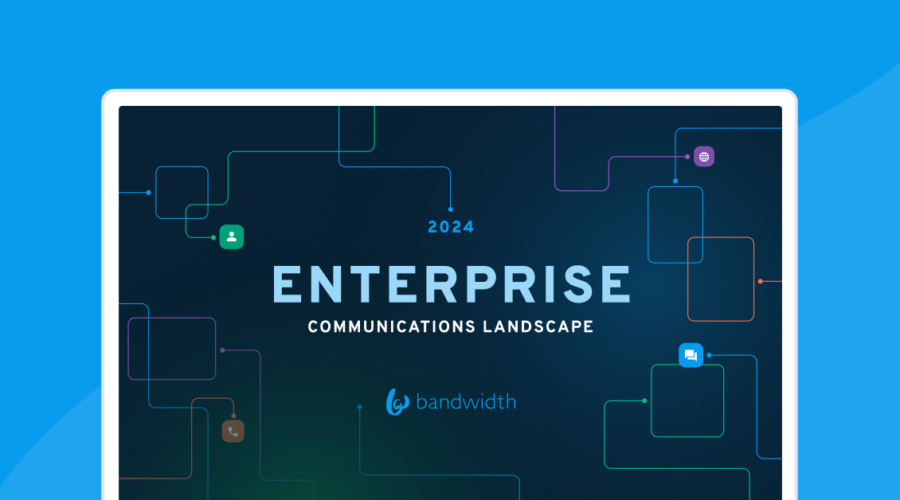
Webinar
Messaging
Bandwidth Messaging AMA
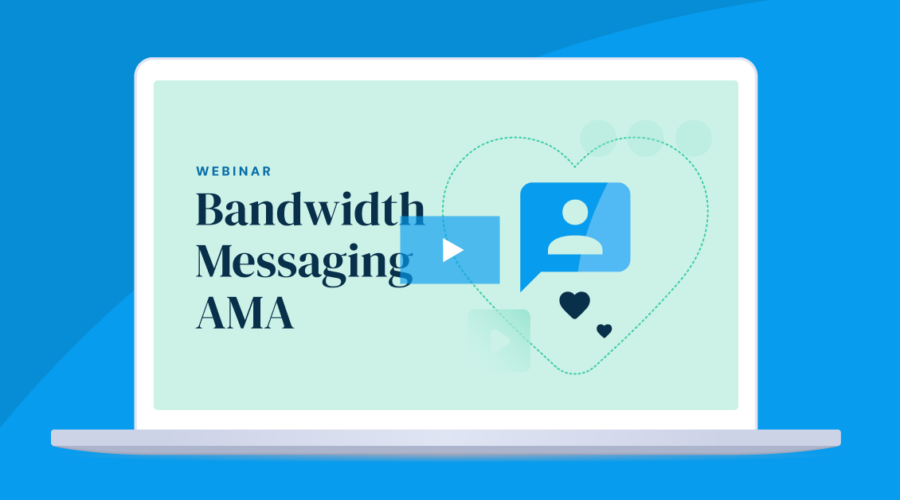
Webinar
Messaging
State of Messaging 2024
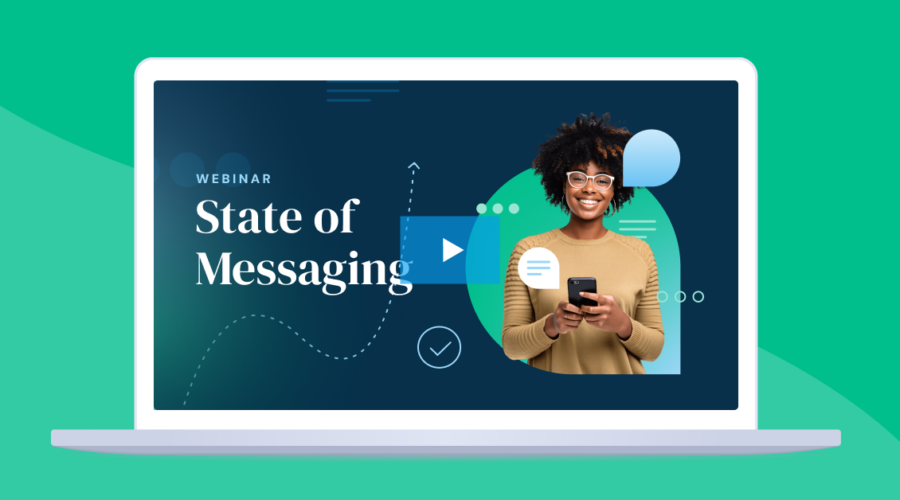
Webinar
Regulations
Bandwidth Buzz: Compliance
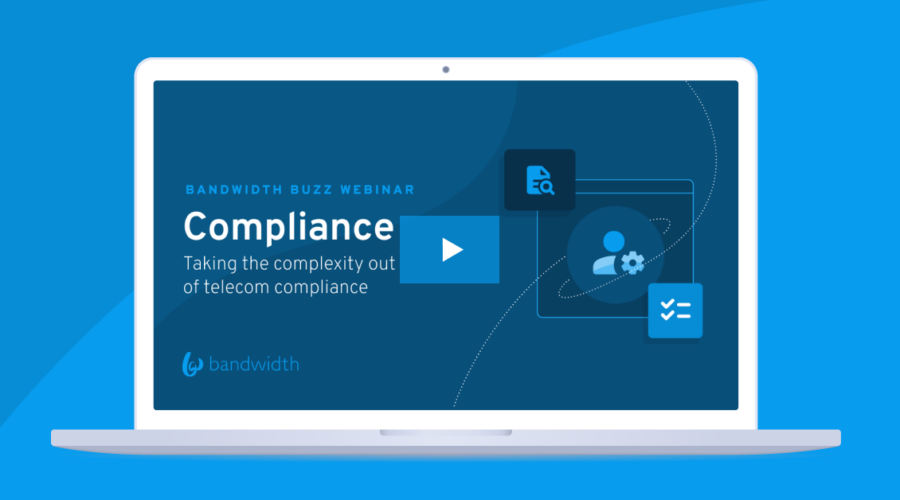
Research Report
Messaging
State of Messaging 2024
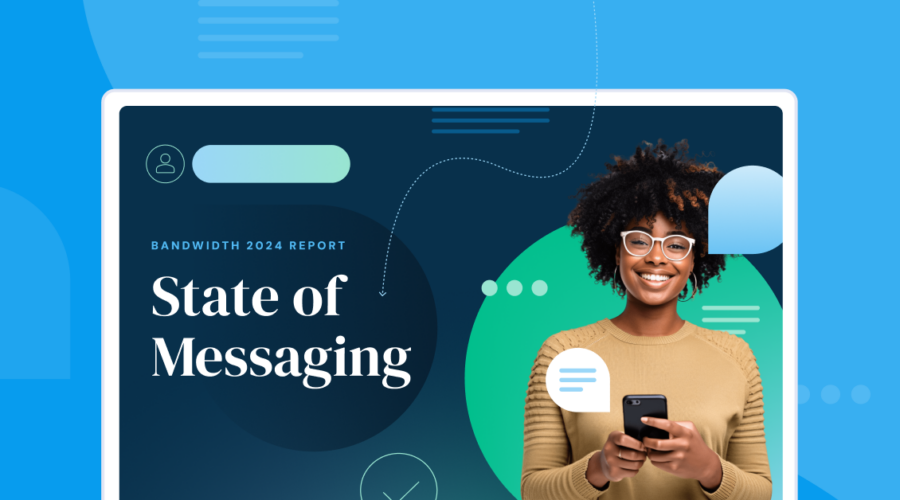
Quick Guide
Messaging
How to Choose Your Messaging Provider
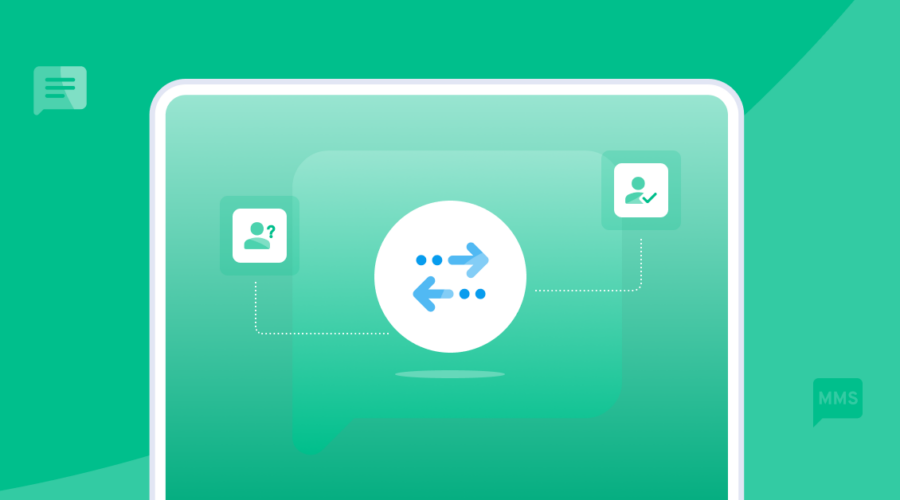
Webinar
Contact Center
AI in Action: How AI is revolutionizing contact center CX
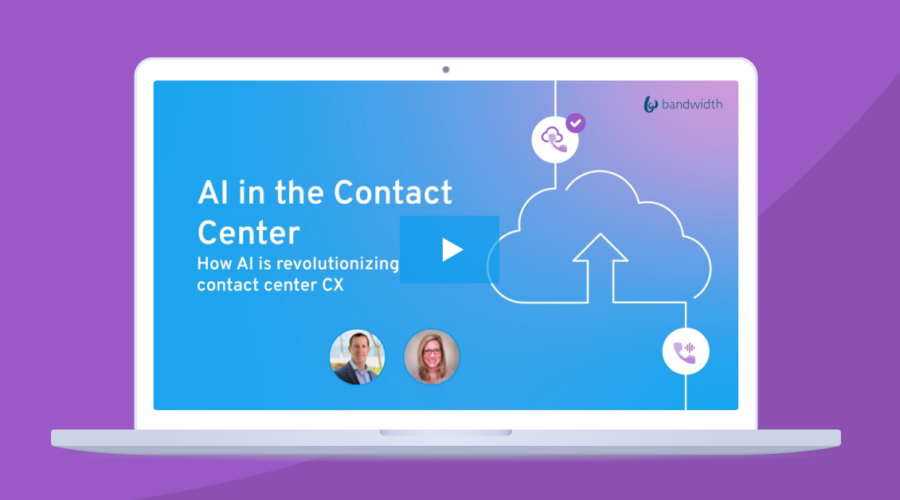
Webinar
Contact Center
Security
Steering your contact center cloud migration to success
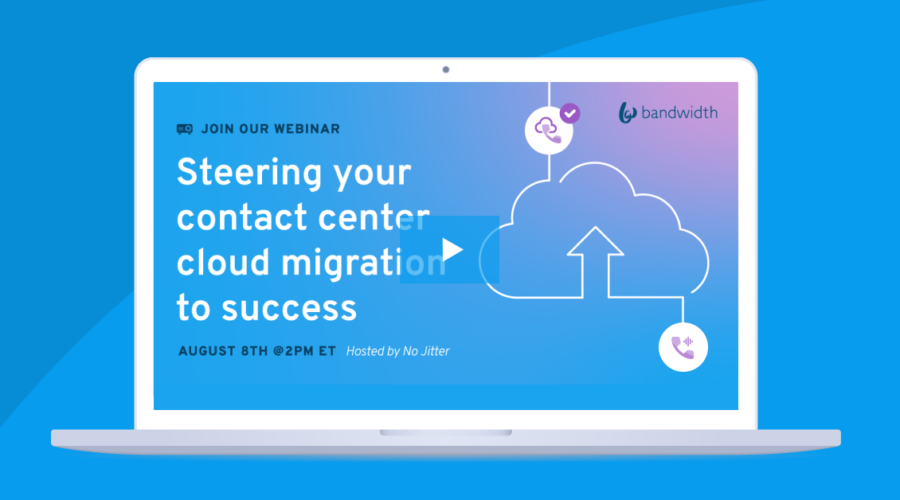
Research Report
Messaging
2023 Patient communication preferences report
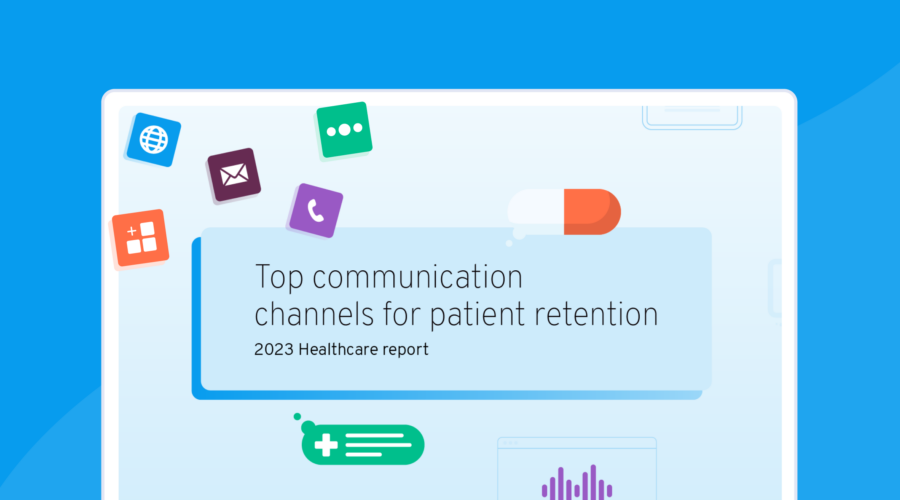